How to Add TDD to an Existing iOS Project
Test-Driven Development (TDD) is one of the best practices of producing clean and maintainabe code base. But if you are integrating TDD in a new project which is being developed in iOS then it is relatively easy but if you are transitioning the project which was not made with TDD approach originally, then it may feel a bit challenging. This guide is meant to be a step-by-step guide to bring TDD into an iOS App if the project is already.
What’s the Use of Going TDD in Legacy Code?
Working on older or existing code and wondering why you should add TDD? Here are a few reasons:
- More Confidence with Refactoring: With tests, you can safely make changes to your code, knowing you won’t accidentally break existing functionality.
- Catching Bugs Early: It is possible to have tests that enable the developers to find out whether there are bugs before they are incorporated into subsequent releases.
- Better Documentation: Your tests also act as documentation, showing how the different parts of your app should behave.
It’s never too late to start using TDD, even if it wasn’t part of the original plan. Here's how you can ease it into your existing iOS project.
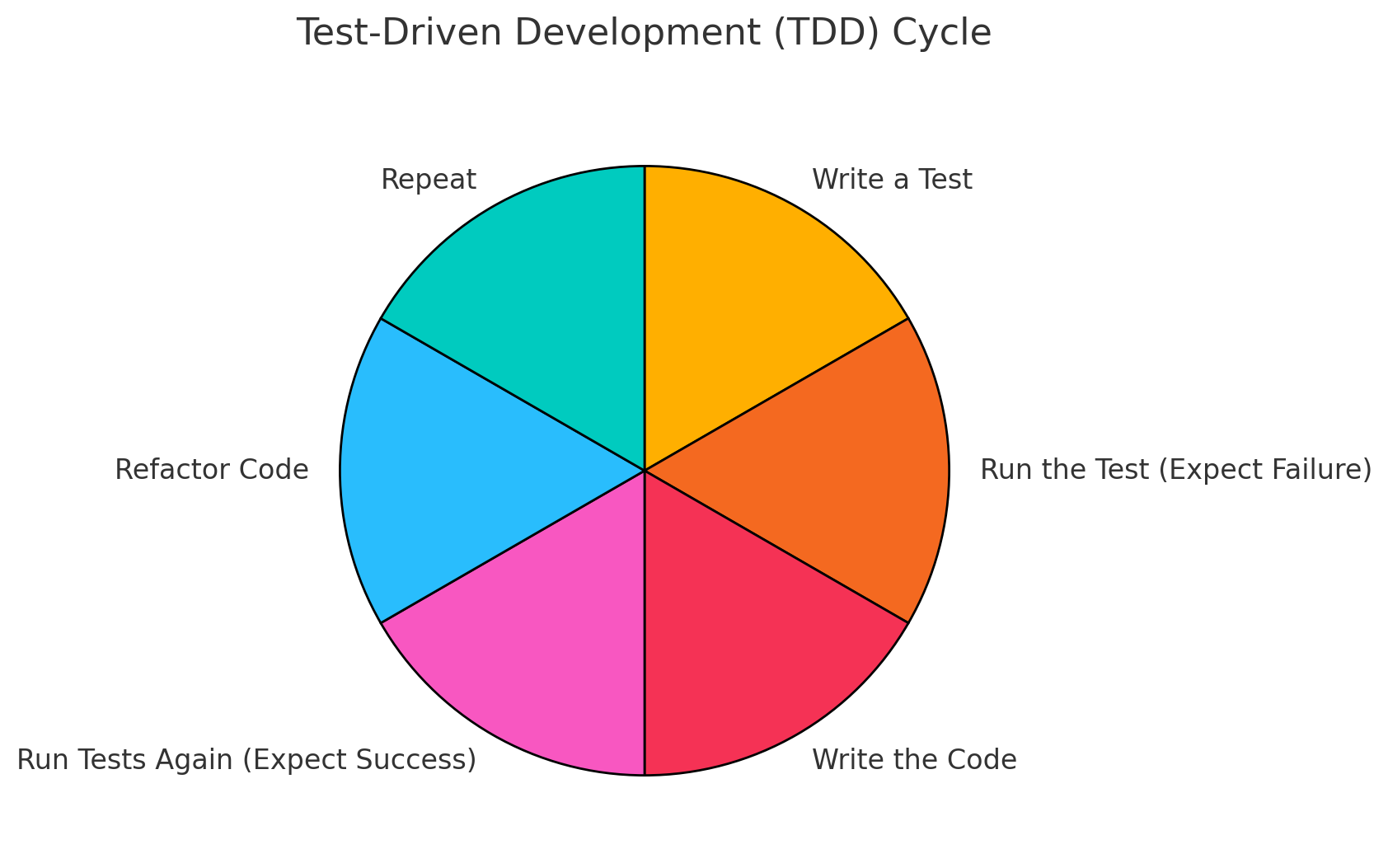
Step-by-Step: Adding TDD to Your Existing Code
1. Start Small: Pick a Simple, Testable Part of Your Code
To start with, choose a Simple, Testable Part of Your Code.Start with something minor such as with a small utility class or creating a simple contiguous method. For instance, in the event you found a method that can reverse a string, this is great as it has insignificant dependency.
Example:
class StringHelper {
func reverse(_ input: String) -> String {
return String(input.reversed())
}
}
2. Write a Test Before You Write or Change Code
In TDD, the first thing you do is write a test that describes what your method should do. Let’s create a test to check that the reverse
method works as expected.
Test Example:
import XCTest
class StringHelperTests: XCTestCase {
func testReverse() {
let stringHelper = StringHelper()
let result = stringHelper.reverse("hello")
XCTAssertEqual(result, "olleh", "The reverse function should reverse the string")
}
}
This simple test makes sure that when you reverse "hello", you get "olleh".
3. Refactor with Confidence
With your test in place, you can now safely refactor your code. If something breaks, your test will tell you immediately.
Let’s say you decide to rewrite the reverse function using a more functional approach:
class StringHelper {
func reverse(_ input: String) -> String {
return input.reduce("") { "\($1)" + $0 }
}
}
After you refactor, run your test again to make sure everything still works. If it passes, great! If not, you know exactly what went wrong.
4. Slowly Add More Tests
Once you’ve got a few tests running for simpler parts of your code, you can move on to more complex sections. Don’t try to test everything at once—take it step by step.
For example, let’s say the next part of your app you want to test is a networking function that builds URL requests. Here’s a small piece of code for that:
class NetworkHelper {
func createRequest(url: String) -> URLRequest? {
guard let url = URL(string: url) else { return nil }
return URLRequest(url: url)
}
}
You can write a test to ensure it works correctly:
func testCreateRequest() {
let networkHelper = NetworkHelper()
let request = networkHelper.createRequest(url: "https://example.com")
XCTAssertNotNil(request, "Request should not be nil")
XCTAssertEqual(request?.url?.absoluteString, "https://example.com")
}
5. Focus on the Most Important Code First
Start by writing tests for the parts of your app that are most critical to your business. These are the areas that would cause the most trouble if they broke—like payment handling or user authentication.
Example: If your app has a login validation function, this would be important to test:
class AuthService {
func isValidCredentials(username: String, password: String) -> Bool {
return !username.isEmpty && password.count >= 8
}
}
You could write tests for that like this:
func testValidCredentials() {
let authService = AuthService()
XCTAssertTrue(authService.isValidCredentials(username: "user", password: "password123"))
XCTAssertFalse(authService.isValidCredentials(username: "", password: "password123"))
XCTAssertFalse(authService.isValidCredentials(username: "user", password: "short"))
}
6. Mock Dependencies When Necessary
In an existing iOS project, you’ll likely have dependencies on things like network requests or databases. You can’t always test these directly, so you might need to use mocks or stubs to simulate these interactions.
For example, let’s say you have a network service that fetches data from an API. You can create a mock version to simulate different outcomes without making actual network calls:
class MockNetworkService: NetworkService {
var shouldReturnError = false
override func fetchData(completion: (Result<Data, Error>) -> Void) {
if shouldReturnError {
completion(.failure(NSError(domain: "", code: 0, userInfo: nil)))
} else {
let mockData = Data() // Simulate successful data response
completion(.success(mockData))
}
}
}
Tips
- Start with Core Logic: Begin testing the parts of your app that are easiest to isolate, like utility methods or business rules.
- Use Xcode’s Code Coverage Tool: This helps you see what’s already covered by tests and where to focus next.
- Don’t Stress About 100% Coverage: While full test coverage is ideal, it’s not always possible with legacy code. Start by testing the most important parts of your app.
- Refactor Gradually: Use tests as a safety net to clean up messy code as you go.
Wrapping Up
While starting to apply TDD in an existing iOS project it may seem rather challenging, but the benefits repay the effort. If you start small, concentrate on important code, and add tests step by step you’d enhance your app’s stability and decrease the number of bugs, therefore gaining confidence when refactoring.
Every test you add increases the likelihood of your app’s success and with time, your codebase will become clean.
Thank you for reading!